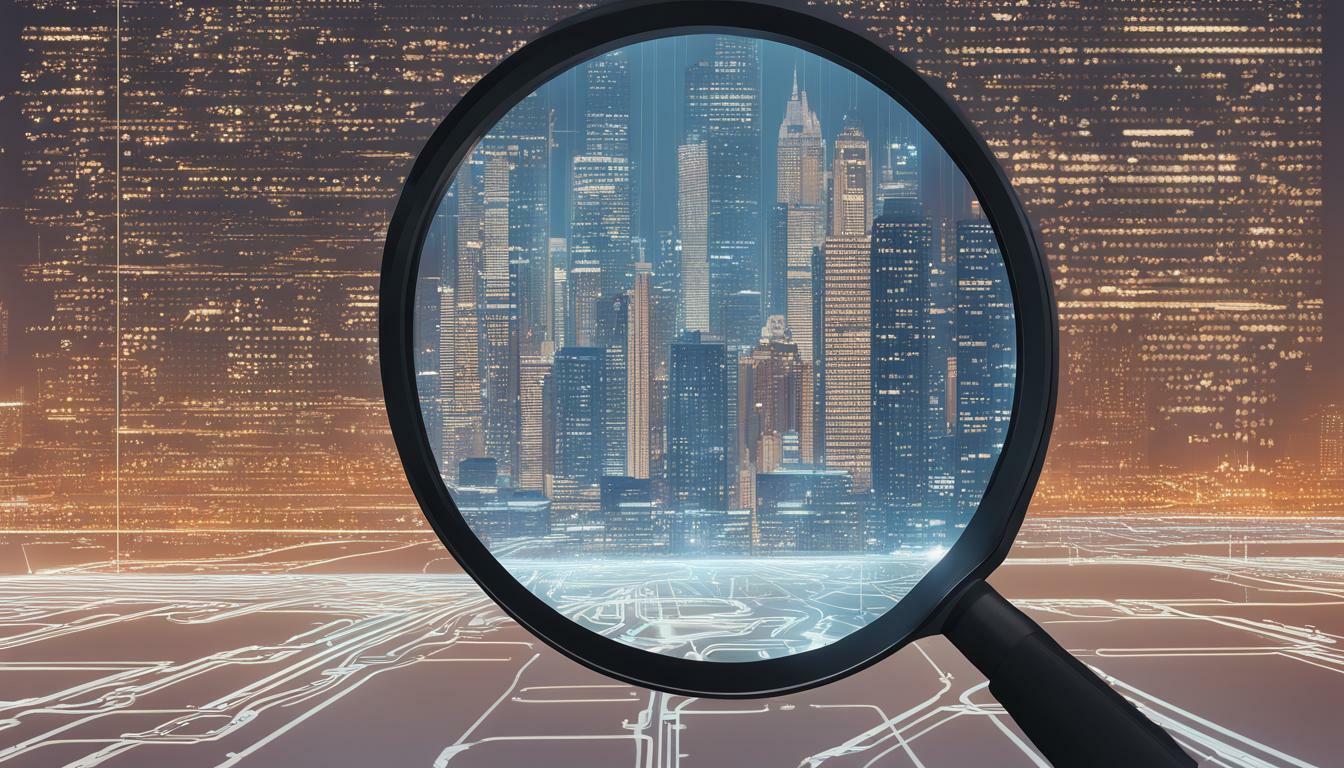
Software design patterns are essential tools that help developers create scalable, maintainable, and reusable code. These patterns have significant real-world applications that can provide solutions to common software development challenges. In this section, we will explore the practical use cases of software design patterns and provide a comprehensive analysis of their importance in software development.
By understanding the fundamental principles of design patterns in software development, developers can leverage them to create solutions that address a wide range of challenges. From performance optimizations to managing complex codebases, well-implemented design patterns can streamline the development process and produce better results.
Key Takeaways
- Software design patterns offer practical solutions to common software development challenges.
- Understanding and implementing design patterns effectively can enhance code quality and streamline the development process.
- Design patterns can help developers create scalable, maintainable, and reusable code.
- Well-implemented design patterns can produce better results and enhance software quality.
Examples of Software Design Patterns in Practice
Software design patterns offer practical solutions to common software development challenges. In this section, we will explore various examples of software design patterns and how they are implemented in real-life scenarios.
Singleton Pattern
The Singleton pattern is used when we need to ensure that only one instance of a class is created. This pattern is commonly used in situations where we need to limit the number of database connections, file handlers, or thread pools.
For example, consider an application that needs to read and write data to a single file. Without using the Singleton pattern, multiple instances of the file handler class could be created, leading to potential inconsistencies in the data.
By implementing the Singleton pattern, we ensure that only one instance of the file handler class is created, and all requests to read or write data are handled by that instance. This results in a more stable and predictable application.
Observer Pattern
The Observer pattern is used when we need to notify multiple objects of a change in state in an object. This pattern is commonly used in graphical interfaces, where multiple objects need to be notified when a user interacts with a specific object.
For example, consider a music player application that displays the current track being played. Using the Observer pattern, we can notify all the user interface objects (such as the track name, artist, album art) to update their display when a new track is played.
By implementing the Observer pattern, we can improve the modularity and maintainability of the application. Instead of tightly coupling the user interface objects with the music player object, we can decouple them and make them more reusable in other parts of the application.
Factory Method Pattern
The Factory Method pattern is used when we need to create objects from a set of related classes. This pattern is commonly used in situations where we need to abstract the creation of objects from the client code.
For example, consider an online store application that sells different types of products. Using the Factory Method pattern, we can create a factory class that abstracts the creation of different product objects (such as books, electronics, toys) from the client code.
By implementing the Factory Method pattern, we can improve the flexibility and scalability of the application. If we need to add new types of products in the future, we can do so without changing the client code, by simply adding a new subclass to the factory class.
These are just a few examples of the many software design patterns available for developers. By understanding and implementing these patterns effectively, developers can improve the quality and maintainability of their code, and create more robust and scalable applications.
Benefits of Implementing Design Patterns
Software design patterns are gaining popularity in the industry for their numerous benefits in software development. By implementing design patterns, developers can achieve better code quality, maintainability, and reusability while reducing development time and costs. Let’s explore the benefits of using design patterns in more detail:
Improved Code Readability
Design patterns are standardized solutions to common coding problems, making code more structured and readable. By using recognizable structures, such as the Template Method or the Observer pattern, developers can easily understand how code functions and ensure consistency across projects.
Enhanced Maintainability
Design patterns are modular and independent, making it easier to modify and maintain code. By separating concerns and isolating functions, developers can ensure that changes or updates to one part of the code will not affect other parts of the system. This also reduces the potential for bugs and errors.
Increased Reusability
Design patterns offer solutions to common problems that can be reused across various projects. By using tested and proven solutions, developers can reduce development time and costs while ensuring code consistency and quality. This also makes it easier for developers to collaborate and work on projects together.
Enhanced Software Quality
Design patterns promote best practices and emphasize the importance of code quality. By using patterns, developers can ensure that code is well-structured, easy to maintain, and scalable. This results in higher quality software that is more reliable and less prone to errors and bugs.
Time-to-Market Reduction
Design patterns promote faster development by providing solutions to common problems. By using standardized structures and solutions, developers can reduce development time and costs, allowing projects to be completed more quickly and efficiently.
Overall, design patterns offer numerous benefits to the software development industry. By understanding and implementing them effectively, developers can improve code quality, maintainability, and reusability while reducing development time and costs. These benefits make design patterns an essential tool for any developer or software development team.
Case Studies on Real-life Implementation of Design Patterns
Real-life implementation of design patterns has become increasingly important in modern software development. The ability to apply design patterns effectively can make a significant difference in project outcomes, such as performance, scalability, and robustness.
Case Study 1: Implementing the Observer Pattern in E-commerce
One excellent example of real-life implementation of design patterns is the Observer pattern. In e-commerce, this pattern is used to notify customers when a product’s price drops or when an item becomes available.
Benefits of Implementing Observer Pattern in E-commerce | Challenges Faced in Implementation |
---|---|
|
|
Despite the challenges faced in implementation, the Observer pattern’s benefits have made it a popular choice among e-commerce websites.
Case Study 2: Implementing the Factory Pattern in Manufacturing
The Factory pattern is a popular design pattern used in the manufacturing industry. It is used to create objects dynamically, based on specific conditions or user requirements.
Benefits of Implementing Factory Pattern in Manufacturing | Challenges Faced in Implementation |
---|---|
|
|
By implementing the Factory pattern, manufacturers have been able to improve product quality and meet customer demands more effectively.
Importance of Understanding Design Patterns
Understanding design patterns is essential for software developers seeking to improve the quality of their code. By familiarizing themselves with existing patterns, developers can choose the most appropriate solution for a given problem. Furthermore, understanding design patterns can help developers collaborate more effectively with their peers, improving communication and reducing project errors.
Overcoming Challenges in Applying Design Patterns
While software design patterns offer practical solutions to common development challenges, applying them can sometimes be challenging. Below are some common obstacles and strategies for overcoming them:
- Choosing the right pattern for the problem: Sometimes, it can be difficult to determine which pattern to use for a specific challenge. One strategy is to thoroughly understand the problem before choosing a pattern. Developers can also consult design pattern catalogs or seek advice from experienced colleagues.
- Adapting patterns to fit specific contexts: Design patterns are not one-size-fits-all solutions. They must be customized to fit specific contexts, which can be challenging. Developers can overcome this challenge by thoroughly analyzing the problem space, identifying the relevant patterns, and modifying them to fit the context.
- Ensuring consistency: When multiple developers work on a project, ensuring consistency in the application of design patterns can be difficult. To overcome this challenge, developers should establish design guidelines and conduct code reviews to ensure adherence to the established patterns.
- Learning curve: Design patterns can have a steep learning curve, which can discourage adoption. To overcome this challenge, developers can start with simple patterns and gradually progress to more complex ones. They can also take advantage of online resources or training programs to improve their understanding.
By understanding and overcoming these challenges, developers can successfully apply design patterns in their projects, enhancing code quality and improving project outcomes.
Conclusion
After a comprehensive analysis of the real-world applications of software design patterns, it is clear that these patterns offer practical solutions to common software development challenges. By understanding and implementing these patterns effectively, developers can enhance code quality and streamline the development process.
The importance of design patterns in software development cannot be overemphasized. They improve code readability, maintainability, and reusability. They also have a significant impact on software quality and time-to-market.
Real-world Applications of Software Design Patterns
The real-world applications of software design patterns are evident in many industries. From e-commerce to healthcare, finance, and more, design patterns have been used to improve system performance, scalability, and robustness.
By applying design patterns effectively, developers can build reliable software systems that can adapt to changes in requirements and business needs. Furthermore, design patterns enable developers to write code that is more efficient, reusable, and maintainable.
In conclusion, understanding and implementing software design patterns is critical for developers who want to build high-quality software systems that meet the demands of modern industries. By incorporating design patterns into their development process, developers can improve code quality, reduce maintenance costs, and deliver better software systems to their clients and customers.
FAQ
Q: What are software design patterns?
A: Software design patterns are reusable solutions to common problems that occur during software development. They provide guidelines and templates for designing software systems that are efficient, maintainable, and scalable.
Q: Why are software design patterns important?
A: Software design patterns are important because they promote best practices and help developers write code that is more readable, maintainable, and reusable. They also provide a common language and framework for communication between developers.
Q: How can software design patterns be implemented in real-world scenarios?
A: Software design patterns can be implemented in real-world scenarios by identifying common problems or challenges in the software development process and applying the appropriate design pattern that offers a solution. By understanding the different types of design patterns and their use cases, developers can effectively solve problems and improve the overall quality of their software.
Q: What are the benefits of implementing software design patterns?
A: Implementing software design patterns offers several benefits, including improved code readability, maintainability, and reusability. Design patterns also help to reduce development time and costs, enhance software quality, and facilitate collaboration among developers.
Q: Can you provide examples of real-life implementation of design patterns?
A: Yes, there are numerous examples of real-life implementation of design patterns across various industries. For example, the Observer pattern is often used in event-driven systems, the Singleton pattern is commonly applied in database connections, and the Strategy pattern can be found in software that requires interchangeable algorithms.
Q: What challenges can arise when applying design patterns?
A: Some common challenges when applying design patterns include choosing the right pattern for a specific problem, understanding the intricacies and trade-offs of different patterns, and ensuring that the pattern is implemented correctly in the software system. However, with proper understanding and experience, these challenges can be overcome.